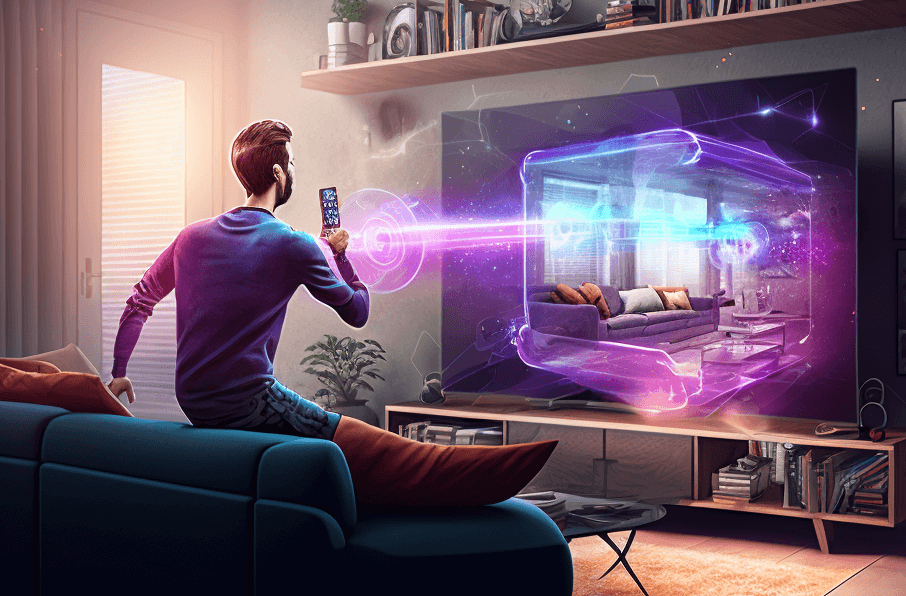
What are pointers and pointer events in smart TV applications
Pointer events, pointers or sometimes mouse and mouse events – there are multiple ways to call this interaction between the user and the TV, but they’re not actually exactly the same. Basically, the pointer (or mouse) is an alternative way to control your TV and applications besides the remote’s normal navigation buttons, and it mostly works in the same way as your mouse works with your computer, by making selections via pointing and clicking. Pointer/mouse events, on the other hand, are the actual events that are fired in the application via interactions like entering elements, clicking them etc.
Which TVs support pointer events
First off, many TV models, for example Sony (Android TV), Samsung and LG, support regular USB computer mice if your TV just has the corresponding port for it. This is a very easy way to test your application’s pointer support quickly, as you can just plug a mouse in and don’t need any other hardware like a specialized remote. Some HbbTV devices also have an option to support pointer events.
Some LG televisions even have support for their own pointer-enabled remote controller called the MRCU (Magic Remote Control Unit), which is a point and click device which triggers the pointer events on the supported and registered TV just like a mouse would.
Examples of possible pointer events
Pointer events that are supported by smart TV platforms are usually the same ones that are used in other web applications. You could, for example, have an element that does something when you hover over it, click it or leave its area. Some usable events in a TV application could be, for example, “click”, “mouseenter”, “mouseleave”, “mouseover”, “mousemove”. If you’ve worked with web applications before, and especially JavaScript, these are probably familiar to you.
Considerations with user interface regarding pointer interactions
Due to pointers being a way of navigation, it is important to take into account the other navigation methods of the application and platform.
For example, if the user has navigated to a certain element in the view using conventional directional navigation and the element is clearly focused, what would you do when a pointer was first hovered over the element and then moved out of it and onto another element? You would probably change the focus to the new element due to the pointer event, and if the user would resume using directional navigation, the focus would probably continue from the latest focused element the pointer has touched.
Mixing and matching navigation methods can make the navigation a bit more complex for the developer, but no element or use case should be out of the end user’s reach because it’s only accessible via pointer. If designing an application with heavy emphasis on the pointer, it’s important to remember that some older platforms might not have that support, and the regular directional navigation should be supported.
Read more about UI/UX design for Smart TV applications on our other blog post https://sofiadigital.com/how-to-design-ui-ux-for-smart-tv-apps/
Development
Starting point
I will be making this development tutorial using my LG TV and building the application on top of our pre-existing project that was started in our earlier blog here: How to handle remote control keys in a Smart TV application?
You can also start developing with LG televisions by following the tutorial on their developer portal: https://webostv.developer.lge.com/develop/getting-started/developer-workflow
This is going to be a quick and easy tutorial where I’ll create a single element that can then listen to a “click” event which is fired when the pointer is hovered on top of the element and the pointer device’s “select” is pressed.
Create element for pointer events
As a first step, we need to create an element that can be used as a point of interaction with the pointer. These elements can usually be anything that your smart TV supports, like buttons, content division, paragraphs etc. Here I’ve created a simple div element with the id “playButton”. This will be our interactable button, and it’s been placed inside the body of the HTML file.
Hello Awesome App!
PLAY
I’ve also added some simple CSS for the div so that it doesn’t get lost in the white background so easily.
#playButton {
display: block;
width: 80px;
height: 60px;
border: 2px solid;
text-align: center;
}
Add pointer event variables
As an example, I’m adding a variable to the start of our file which will later store our pointer event names. This is just in case, so if we have multiple elements with the same pointer event, we wouldn’t need to rewrite the event name again and again. This is just for the click event, but here you could also have the “mouseenter”, “mouseleave” etc.
/**
* Pointer click event variable
*/
let POINTER_CLICK;
Register pointer events
Now that we have our pointer event variables, we can register some actual events to them by giving them the event names that are actually used by the event listeners.
/**
* Setting pointer event variables so you don't need to rewrite event names for multiple elements
*/
function registerPointerEvents() {
console.log("registerPointerEvents is called");
POINTER_CLICK = "click";
}
Functions for pointer events
Before we can make use of our pointer event and attach a listener to our element, we’re going to need some functionality that the event listener can actually call, so I’ll make a simple function that will change what we’ve printed on the screen to reflect the event that has happened.
/**
* Function which is called when a pointer click event occurs
*/
function pointerClick(event) {
console.log("Pointer click event happened: ", event);
var playButton = document.getElementById("playButton");
if(event.target.id === playButton.id) {
root.innerHTML = "PLAY BUTTON CLICKED";
} else {
// Clear the message that's currently displayed
root.innerHTML = "";
}
}
Add event listener for pointer events and call for the register function
As a last step before testing, we still need to attach the event listener to our element and actually call the register function where we set the value for our POINTER_CLICK variable. The event listener is the actual component that captures the events happening in our application and when triggered will in turn call our previously created function.
/**
* Function for creating the application and getting it ready for the users to use
*/
function createApp() {
console.log("Creating the app...");
registerKeys();
registerPointerEvents();
/**
* Setting the root HTML element
*/
root = document.getElementById("app");
document.addEventListener("keydown", handleKeyPress);
document.addEventListener(POINTER_CLICK, pointerClick);
/**
* Here you can start doing awesome things!
*/
console.log("...and it's ready for use");
}
As I stated in the beginning of this blog tutorial, I’ve built these pointer events on top of our previous navigation blog post, so there’s also some of our old code mixed in there.
I’ve built the mouse handling with LG televisions in mind, so that’s what I’m going to use to test the functionality. If you’ve done the same, you just need to create the required appinfo.json file, and then you can use, for example, Visual Studio Code in conjunction with the “webOS TV” extension to launch your application on an LG device. Let’s see what we’ve got!
Clicking the “Play” button with the pointer has successfully printed out our desired text, and clicking elsewhere doesn’t trigger this effect.
Thank you for reading this simple tutorial regarding pointer events in the Smart TV realm. Like with the previous part, nothing Smart TV specific yet, but we’re getting there!
ABOUT AUTHOR
I am a software developer at Sofia Digital focusing on Smart TV application development. I’ve worked with different platforms and projects for approximately 5 years including Smart TV applications, web and sports watches.