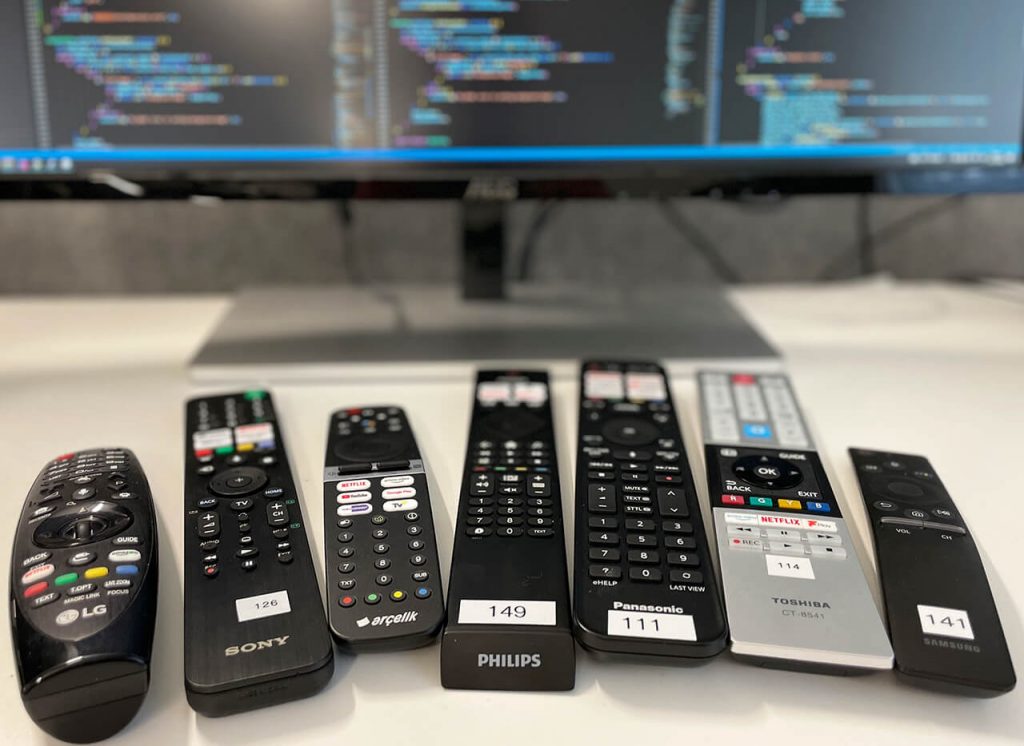
By Janne Lahtela
LET’S GET STARTED!
Let’s start by creating variables for the remote control key codes in our app.js file.
/**
* A remote control key variables
*/
let RC_LEFT,RC_UP,RC_RIGHT,RC_DOWN,RC_ENTER,RC_BACK,RC_EXIT;
These are so that we don’t have to remember what the key codes are later, we just have to remember the variable names.
Next, it’s good to define and store our root element in the application when the application is created.
/**
* The root html element
*/
let root = null;
We will probably have to refer to this at some point when we write our application.
After these, we start creating essential functions for our application, and one of those functions is something which sets the key variable values that we defined earlier. Let’s call it the registerKeys function.
/**
* Setting key event numeric value to variables so you don't have to numeric values
*/
function registerKeys() {
console.log("registerKeys is called");
RC_LEFT = 37;
RC_UP = 38;
RC_RIGHT = 39;
RC_DOWN = 40;
RC_ENTER = 13;
RC_BACK = 10009;
RC_EXIT = 10182;
}
The numeric value here is the code for the key. For example, the Enter key on your keyboard is the same as the Enter key on the remote control. You can find these values on the web or catch them in the application.
At this point, it might be good to define the functions for the key presses before we start combining everything into application form.
/**
* The key press functions
*/
function keyPressUp() {
console.log("You pressed the up key");
root.innerHTML = "UP";
}
function keyPressDown() {
console.log("You pressed the down key");
root.innerHTML = "DOWN";
}
function keyPressLeft() {
console.log("You pressed the left key");
root.innerHTML = "LEFT";
}
function keyPressRight() {
console.log("You pressed the right key");
root.innerHTML = "RIGHT";
}
function selectItem() {
console.log("You pressed the enter key");
root.innerHTML = "ENTER";
}
Nothing too fancy here. As you can see, we’re calling the root element here, so we have to define the element before the application is ready for use. In this simple example, we put the text, e.g. “ENTER”, inside the root element.
Good. Now we have the key codes set and the functions for them. The next step would be something that defines what we do when a key on the remote control is pressed. A switch-case is a good and easy way to separate the keys pressed.
/**
* Function which is called when a key is pressed
*/
function handleKeyPress(event) {
switch(event.keyCode) {
case RC_ENTER:
selectItem();
break;
case RC_EXIT:
break;
case RC_LEFT:
keyPressLeft();
break;
case RC_RIGHT:
keyPressRight();
break;
case RC_UP:
keyPressUp();
break;
case RC_DOWN:
keyPressDown();
break;
default:
console.log("You need to add some cases here, a key you pressed was", event.keyCode);
}
}
When a key is pressed, the switch-case catches the value and executes the case based on that value. The default at the end of the switch-case cases catches the value if it’s not defined yet.
Now we have all the components for the perfect application where key presses are handled. Let’s put everything together and create the app here.
/**
* Function for creating the application and getting it ready for the users to use
*/
function createApp() {
console.log("Creating the app...");
registerKeys();
/**
* Setting the root HTML element
*/
root = document.getElementById("app");
document.addEventListener("keydown", handleKeyPress);
/**
* Here you can start doing awesome things!
*/
console.log("...and it's ready for use");
}
First, we register all the key event codes. After that, we tell the application what our root element is on the index page. Finally, we create an event listener and bind our function which handles the key presses.
If you want to check how your app.js file should look like, check here: download app.js
Now it’s time for the changes in index.html, and because we are creating a larger application, let’s organize the files a bit. Let’s move our app.js to a new “js” folder because it’s possible that we’ll need more JavaScript files in the future.
My Awesome Smart-TV -app!
Hello Awesome App!
What are we doing here? Why are we using an event listener here? The answer: We want to be sure that the HTML page is ready before we start doing anything. After the HTML page is ready, we call our CreateApp-function, which is in the app.js file.
Now, if you’re using Tizen Studio to launch your app, copy the files into the Tizen project, connect the device (mine is a Samsung 2020 model) and launch the app!
When the app is running, press the keys we defined earlier, and you should see the text we defined earlier.
This is a simple example of how to handle key press events in a Smart TV application. From the coding perspective, we haven’t done anything Smart TV platform specific yet.
What should you learn next?
Next steps:
- Learn what mouse events are and where you need those.
- Learn how you can implement mouse events in your Smart TV application.
- Learn what is object-oriented programming and why you need that in Smart TV development.
Actually we have a blog post coming up about mouse events in Smart TV development. Subscribe to our newsletter or follow us in LinkedIn / Twitter to get the latest updates!
About author
I am currently working at Sofia Digital Ltd as a Scrum Master and software developer. I have four years of experience in Smart TV application development and over 20 in web development.